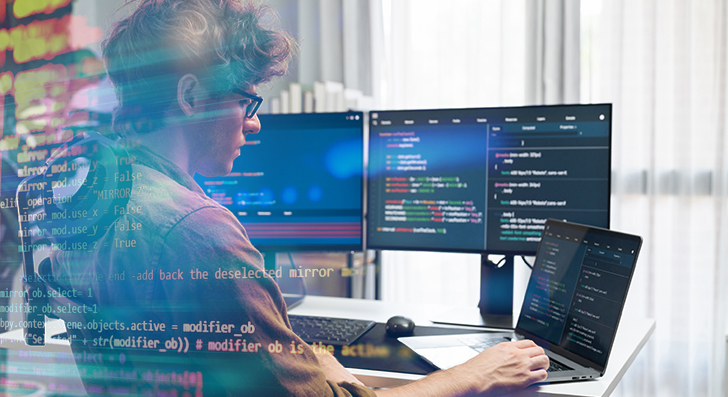
Scalability implies your software can take care of development—more buyers, additional info, and even more visitors—without breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and realistic guidebook to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it ought to be portion of your system from the beginning. A lot of applications fall short when they increase quick mainly because the original style and design can’t deal with the additional load. To be a developer, you should Imagine early about how your method will behave stressed.
Start by planning your architecture to generally be flexible. Prevent monolithic codebases where almost everything is tightly related. As a substitute, use modular style or microservices. These designs crack your application into smaller, impartial sections. Each module or support can scale By itself without having impacting The complete system.
Also, take into consideration your databases from working day a person. Will it require to deal with 1,000,000 users or perhaps a hundred? Select the suitable style—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t want them nevertheless.
A different vital point is to avoid hardcoding assumptions. Don’t create code that only operates beneath present problems. Think about what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like message queues or occasion-driven methods. These aid your app manage far more requests with no receiving overloaded.
If you Establish with scalability in mind, you're not just preparing for success—you are decreasing foreseeable future complications. A properly-planned method is less complicated to take care of, adapt, and increase. It’s greater to organize early than to rebuild later.
Use the proper Database
Deciding on the suitable databases is often a crucial A part of building scalable purposes. Not all databases are created precisely the same, and using the Incorrect you can sluggish you down or maybe lead to failures as your app grows.
Get started by knowledge your info. Is it remarkably structured, like rows within a desk? If Indeed, a relational database like PostgreSQL or MySQL is a good healthy. They're strong with associations, transactions, and consistency. Additionally they assistance scaling approaches like go through replicas, indexing, and partitioning to take care of additional site visitors and details.
When your data is much more adaptable—like user action logs, product catalogs, or paperwork—consider a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured facts and can scale horizontally a lot more conveniently.
Also, contemplate your examine and create designs. Are you presently undertaking lots of reads with fewer writes? Use caching and browse replicas. Are you presently handling a weighty generate load? Consider databases that could tackle high produce throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term info streams).
It’s also clever to Imagine ahead. You may not require Highly developed scaling attributes now, but selecting a database that supports them implies you gained’t need to have to modify afterwards.
Use indexing to hurry up queries. Stay clear of avoidable joins. Normalize or denormalize your data dependant upon your entry designs. And generally watch databases general performance when you mature.
To put it briefly, the ideal databases relies on your app’s structure, velocity requires, And exactly how you hope it to improve. Acquire time to select correctly—it’ll preserve plenty of problems later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller hold off adds up. Poorly penned code or unoptimized queries can decelerate effectiveness and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Start by crafting cleanse, straightforward code. Steer clear of repeating logic and take away nearly anything unneeded. Don’t choose the most complicated Alternative if an easy a single works. Keep the features short, centered, and easy to check. Use profiling equipment to find bottlenecks—sites wherever your code normally takes too very long to run or takes advantage of excessive memory.
Subsequent, evaluate your database queries. These normally sluggish matters down a lot more than the code itself. Be certain Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and as an alternative pick out particular fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, In particular across huge tables.
For those who discover the exact same data currently being asked for again and again, use caching. Retailer the results briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your app a lot more successful.
Make sure to exam with large datasets. Code and queries that perform wonderful with a hundred documents could possibly crash once they have to deal with 1 million.
In a nutshell, scalable apps are speedy applications. Keep the code limited, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with additional people plus more targeted visitors. If everything goes through one server, it'll quickly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments enable maintain your application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server accomplishing the many operate, the load balancer routes end users to distinct servers according to availability. This means no one server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it might be reused speedily. When customers ask for precisely the same data once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. It is possible to serve it through the cache.
There are two prevalent varieties of caching:
one. Server-aspect caching (like Redis or Memcached) retailers details in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances speed, and can make your application additional effective.
Use caching for things which don’t change typically. And always be sure your cache is current when info does transform.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app cope with a lot more buyers, stay rapidly, and Get better from problems. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To build scalable programs, you may need instruments that permit your app develop conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess potential potential. When targeted visitors increases, you can add much more sources with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also provide providers like managed databases, storage, load balancing, and safety tools. You are able to concentrate on constructing your app rather than managing infrastructure.
Containers are another vital Software. A container deals your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, out of your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it automatically.
Containers also help it become simple to independent aspects of your app into services. You may update or scale elements independently, which is perfect for performance and trustworthiness.
In brief, working with cloud and container resources usually means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your application to develop devoid of limits, start out using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Watch Every thing
In case you don’t observe your application, you gained’t know when points go wrong. Monitoring allows you see how your app is doing, location issues early, and make much better selections as your application grows. It’s a vital part of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and expert services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic will help you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Put in place alerts for significant challenges. One example is, If the reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified quickly. This aids you resolve problems quick, often right before buyers even detect.
Monitoring can also be useful after you make improvements. When you deploy a fresh function and find out a spike in glitches or slowdowns, it is possible to roll it back right before it will cause authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without checking, you’ll skip indications of problems till it’s far too late. But with the best tools in position, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works perfectly, even under pressure.
Closing Ideas
Scalability isn’t only for big firms. Even little applications need a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you could Develop applications that grow easily devoid of breaking more info under pressure. Commence compact, Believe massive, and Establish intelligent.